Thruster: Scaling Bar UI
Objective: Learn ho implement a Scaling Bar for our Thruster UI.
Our player has the option to use his thruster to move faster, but we never implemented a way for his thruster to run out of fuel. So we’re going to implement not only a way to limit our player usage of the Thruster System, but also a way for him to see his current fuel.
The way our system is going to work is simple, the player will start with his fuel tank full, and as he uses his Thruster, his fuel goes down and does not regenerate. Once completely empty, he cannot use the Thruster, but his fuel slowly regenerates until its fuel, allowing him to use it once again.

Lets now make sure we delete our Handle Slide Area, since we’re not going to use, and we’re also going to make sure that our Fill Area and Fill both have no numbers (only zeroes) in their Rect Transform component.
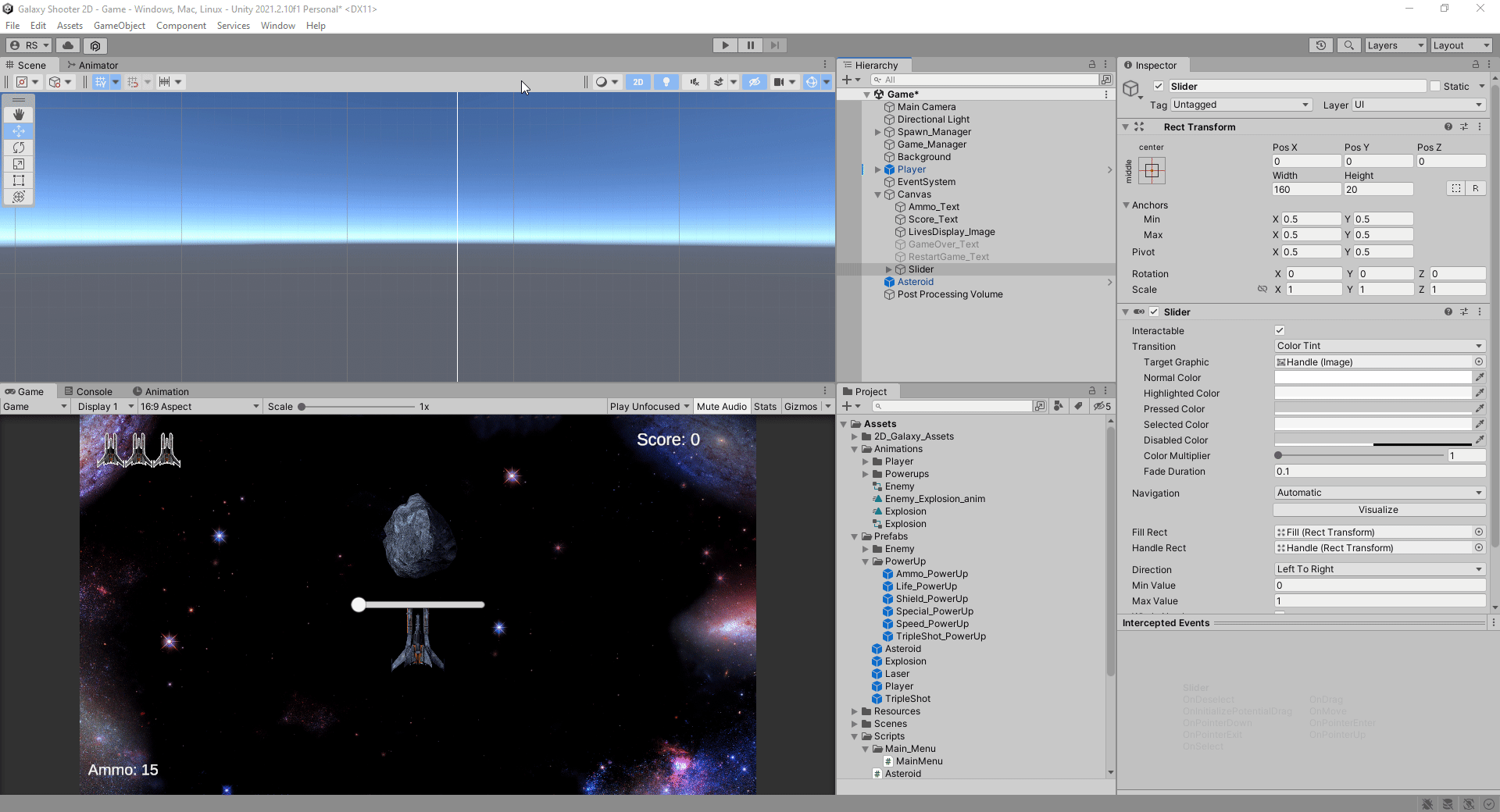
What this does is set the fill are and the fill itself to the full width of the slider.

The change of color makes it easier for us to see the fill for our bar.

Noticed that I also repositioned our bar to be underneath our Lives, making easier for the player to see their UI.

This makes it easier for us to use, since the value itself is the percentage of fuel our player currently has.
We now have our visuals all set up. Let’s proceed with coding it. Since this is related to the UI, we should use our UIManager to handle it.
[SerializeField] private Slider _boostSlider;
private float _boostSliderMaxValue = 100f;
private bool _canUseTurbo = true;
private bool _losingFuel = false;
private bool _gainingFuel = false;
With these variables, we can handle our Slider, we can set our default fuel to 100%, we allow our player the usage of the Thruster System, and we check if we are gaining or losing fuel. In our system, when the player runs out of fuel, it needs to fully recharge before he can use it again.

private void Start()
{
_boostSlider.value = _boostSliderMaxValue;
}
By doing this, we allow the player to have full fuel since the start of the game.
public IEnumerator ThrusterBoostSliderDownRoutine()
{
if (_losingFuel == true)
yield break;
_losingFuel = true;
_gainingFuel = false;
while (_losingFuel == true)
{
_boostSlider.value -= 1f;
yield return new WaitForSeconds(0.05f);
if (_boostSlider.value <= 0f)
{
_boostSlider.value = 0f;
_canUseBoost = false;
_losingFuel = false;
}
}
}
This is the first of the two Coroutines we are going to use. This one drains our fuel when we use our Thruster System.
The first thing we are doing, is preventing from running more than one Coroutine of this type, by checking if we are already losing fuel, and if we are, we just leave.
Now, the first its called, we are neither losing or gaining fuel. So we set our losing fuel to true, so we can go inside our Loop. While we are losing fuel, we gradually reduce the value of our slider, and add a Yield Return to wait a bit before doing it again. We always check if we ran out of fuel, and if that is the case, we can no longer use the boost, and we are no longer losing fuel.
public IEnumerator ThrusterBoostSliderUpRoutine()
{
if (_gainingFuel == true)
yield break;
_gainingFuel = true;
_losingFuel = false;
while (_gainingFuel == true && _canUseBoost == false)
{
_boostSlider.value += 0.75f;
yield return new WaitForSeconds(0.05f);
if (_boostSlider.value == _boostSliderMaxValue)
{
_canUseBoost = true;
_gainingFuel = false;
}
}
}
This one does pretty much the opposite. Again we check if we are already gaining fuel, and if that is the case, we just exit the Coroutine early.
We then set our gaining fuel to true and our losing fuel to false, to prevent the player from losing while he is supposed to be gaining.
Then we go inside our loop. This time we check if we are gaining fuel and if we ran out of fuel, which is controlled by our _canUseBoost variable being false. If that is the case, we slowly grant the player fuel back, and we add a Yield Return to make the process gradual. Once we are full, which means our Slider.value is the same as our _boostSliderMaxValue, we allow the player to use the Thruster System again, and are no longer gaining fuel.
One last thing before we move to our player, our _canUseBoost variable is private, to prevent other script from changing its value. The player needs to know if he can use the Thruster, so we are going to create a method to expose the variable to the player.
public bool CanUseThrusterBoost()
{
return _canUseBoost;
}
Now we go to our Player. Inside the Update method, we are checking if the player is currently holding the LeftShift key to activate the Thruster. This is where we are going to make call of our Coroutines.
if (Input.GetKey(KeyCode.LeftShift) && _uiManager.CanUseThrusterBoost() == true)
{
_isThrusterActive = true;
_thruster.SetActive(true);
StartCoroutine(_uiManager.ThrusterBoostSliderDownRoutine());
}
else
{
_isThrusterActive = false;
_thruster.SetActive(false);
StartCoroutine(_uiManager.ThrusterBoostSliderUpRoutine());
}
So what are we doing on the code above ? Well, first we check if the player is holding the LeftShift key, and he can use the Thruster System.
If he can, we activate our system and start the Coroutine to reduce the value of the slider. This is where adding our variable for checking if we are losing fuel is used. This Coroutine will be started multiple times, since we are in the player’s Update method. Now, when our player reaches the end of the slider, our CanUseThrusterBoost method will return false instead of true, then we reach our else statement. This also occurs when he releases the LeftShift key.
When the player can no longer use the Thruster System, which means our CanUseThrusterBoost is return false, we now start our Coroutine to gradually increase the value of our Slider. Once it reaches the full capacity, our CanUseThrusterBoost will return true again, allowing us to use the system once more.
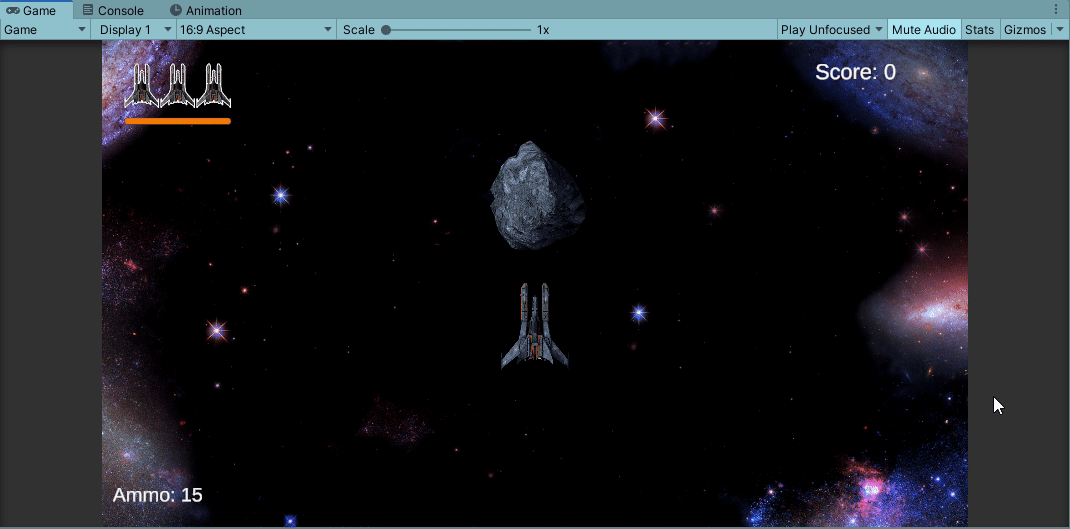
As we can see, as I hold the LeftShift our fuel gradually decreases until its completely depleted. Once its depleted, we just gradually grant it back until its full. Once full, I can use it again. The system works !