Loading Scenes in Unity
Objective: Learn how to load scenes in Unity.
Now we want to give the player an option to Reset the game after it dies, by pressing the R key. This means we have to learn how to Load Scenes in Unity to do this. First we’ll start by giving the player the text tooltip that will be displayed on the screen along with our GameOver text.
[SerializeField] private TextMeshProUGUI _restartGameText;
We can assign the text in the inspector. When activating our GameOver method, we can simply activate this new text as well.
private void ActivateGameOver()
{
_gameOverText.gameObject.SetActive(true);
_restartGameText.gameObject.SetActive(true);
StartCoroutine(GameOverFlickerRoutine());
}
But how do implement such functionality ? How do we restart our game ?
Unity has a SceneManagement library, which allows us to Load scenes.
But who should handle such information ? It doesn’t make sense for our UIManager, in this case our Canvas, to hold information that is related to the state of the game. And also, our player is currently dead, meaning we can’t have this functionality implemented on the player as well.
public class GameManager : MonoBehaviour
{
private bool _isGameOver = false;
private void Update()
{
if (Input.GetKeyDown(KeyCode.R) && _isGameOver)
{
SceneManager.LoadScene(0);
}
}
public void GameOver()
{
_isGameOver = true;
}
}
We created a new class, that will handle all the information regarding the state of the game, such as if the game is currently over or not.
When the game is over, we turn our bool so we know its over, and in our Update method we can call our LoadScene.
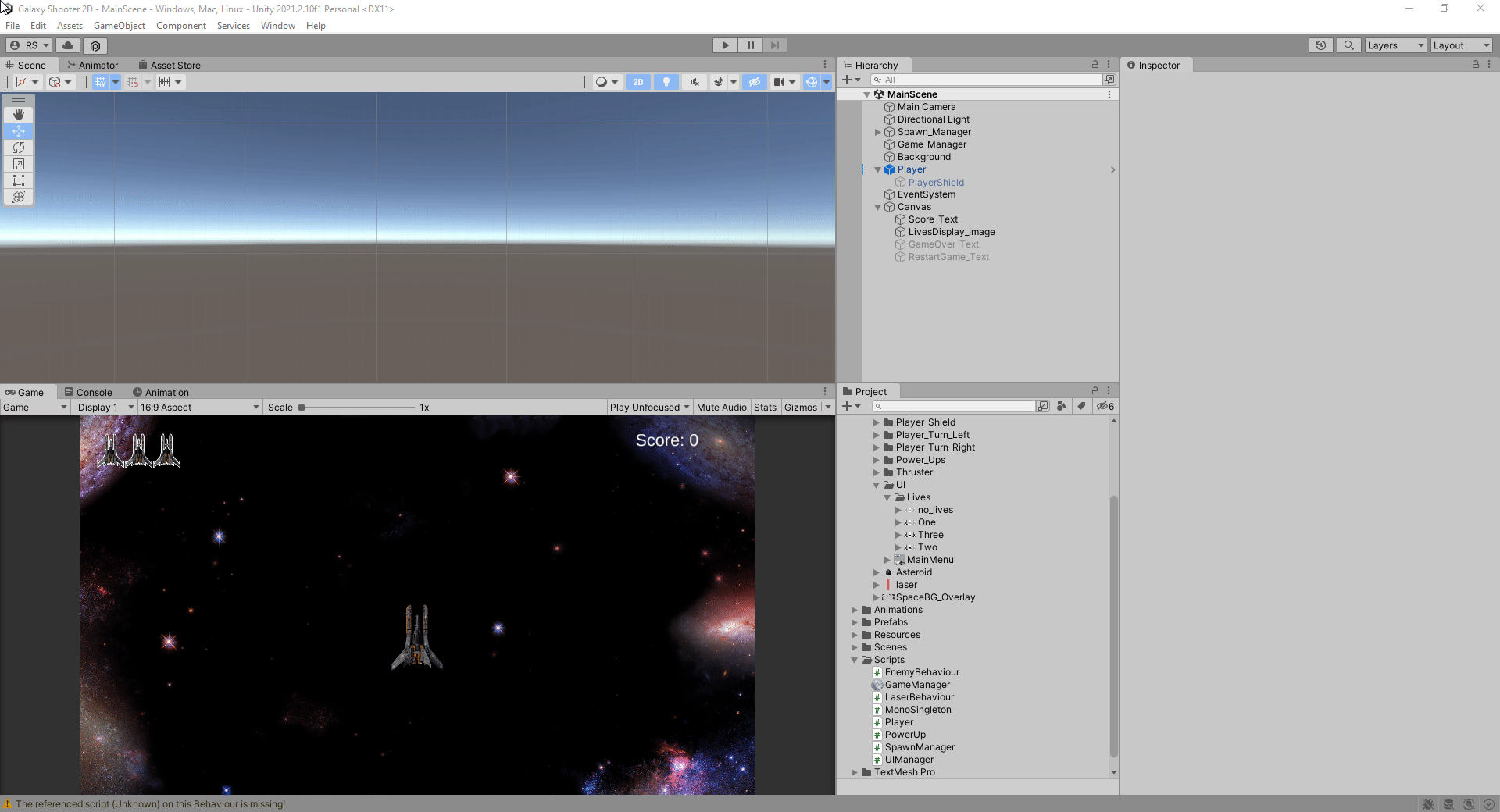
We can see that we have one scene, that is named “Main Scene” and has the index 0.
Inside our GameManager, when our game is over, we wait for player input and reload our game the R key is pressed.
SceneManager.LoadScene(0);
This line loads a specific scene that was passed as parameter. We can use the name of the Scene, which would be “Main Scene”, or provide the integer value of its index, which as we saw in our build settings, is zero.
Integer values are faster than strings, so that is why we are using it instead.
Now we have our functionality to restart, but how do we tell the GameManager that the game is over ?
Our UIManager can tell it, since it knows when we are activating our GameOver UI.
private GameManager _gameManager;
private void Start()
{
_gameManager = GameObject.Find("GameManager").GetComponent<GameManager>();
}
Now we created a variable that will store our GameManager for our UIManager to have access to its script. Now we just need to call the GameOver method from our GameManager when we start our GameOver.
private void ActivateGameOver()
{
_gameOverText.gameObject.SetActive(true);
_restartGameText.gameObject.SetActive(true);
StartCoroutine(GameOverFlickerRoutine());
_gameManager.GameOver();
}

Now we can restart our game after running out of lives!
Let’s implement a Main Menu screen for our game, so when it restarts we go back to our Title Screen instead.
First we can start by creating a new Scene. We are going to name it Main_Menu. I’ve also rename our previous Scene to Game instead of MainScene.

Now we are going to create the image for our MainMenu screen.

Now we have to make a few changes to our image, to make sure it has the right size and position.

Even though we fixed everything in our image, we can see that the background is still too bright for it. Let’s fix it by removing the color.

We’re also going to add our Nebula background to the Main Menu, giving it a proper space vibe.

After having the visuals all set, we are going to create a button, which will be our New Game button, that will start our game.

This button will be responsible for starting our game, so in order for us do to that, we need to create a new script for our MainMenuScene. This script will be attached to the Canvas, as its responsible for the functionality of the button already.
Now in order for us to be able to load our scenes, we are going to manage all scenes in our project, by going to our Build Settings.
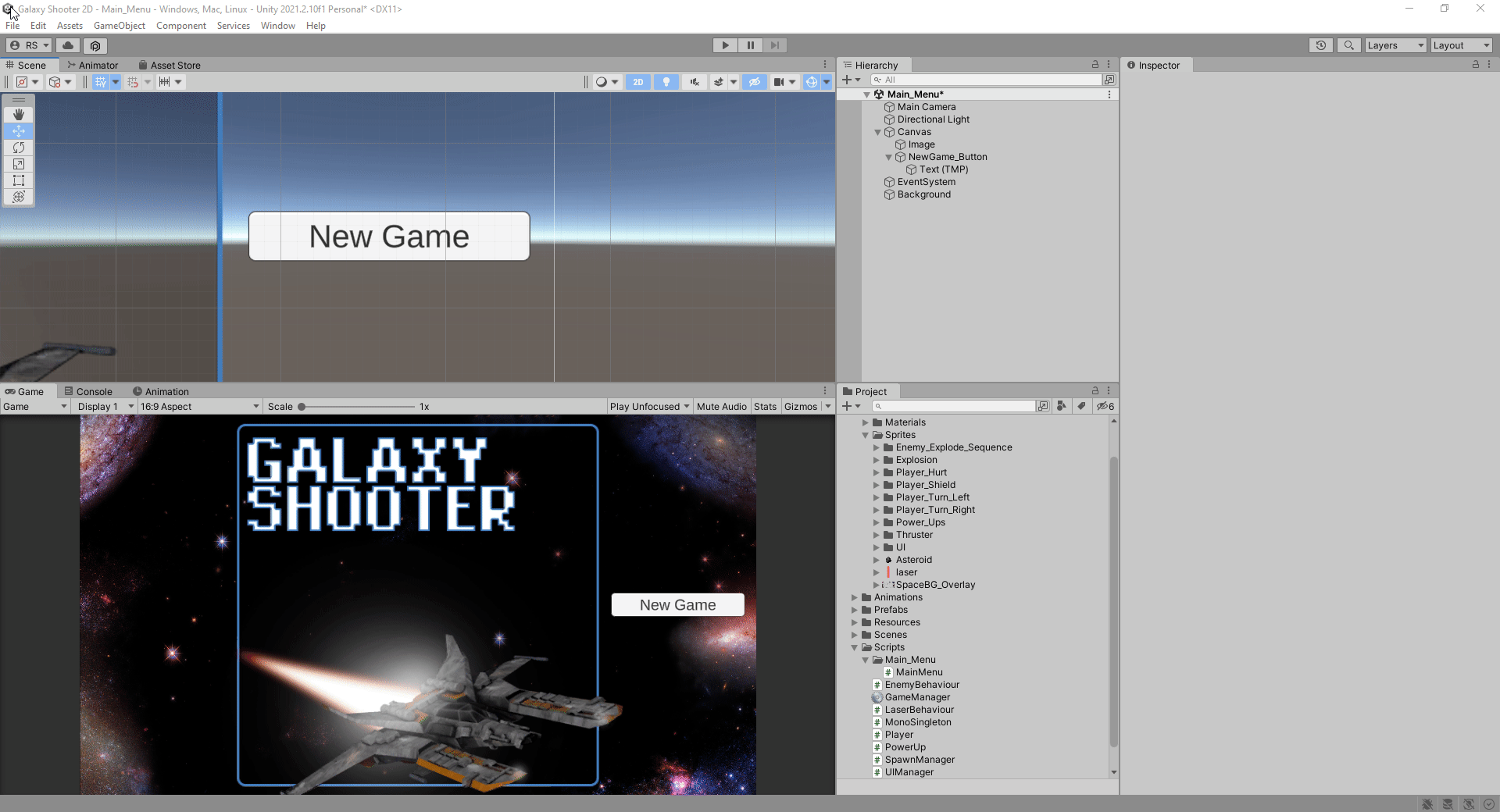
We just placed all scenes in our build, and we now know that our MainMenu is currently our index 0, and the Game is index 1.
public class MainMenu : MonoBehaviour
{
public void LoadGame()
{
SceneManager.LoadScene(1); //Load the Game Scene
}
}
Now we create the method that will load the Game scene when the button is pressed, and then we assign it to the button.

Now that we have our method properly assigned, we just gotta make sure to update our GameManager in our Game scene to load the Game instead of our main menu.
private void Update()
{
if (Input.GetKeyDown(KeyCode.R) && _isGameOver)
{
SceneManager.LoadScene(1); //Load the Game Scene
}
}

Now we have a Main Menu functionality in our game!