Enemy Avoid Shot
Objective: Learn to to implement a new enemy that tries to avoid being hit by our player’s shot.
Again with a new enemy idea for us to try. Lets make a new enemy type, this one is going to try and avoid being hit by our lasers when we fire against him.
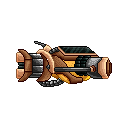
First we have to make sure that our new enemy typeexists in our game.
private enum EnemyType
{
Default,
Zigzag,
Aggressive,
Smart,
Evasive
}
Since this enemy is going to look for our player lasers, we have to make sure everything is set in our SpawnManager and our container for lasers.
[SerializeField] private GameObject _playerLaserContainer;
public GameObject PlayerLaserContainer => _playerLaserContainer;
All we’re doing is creating our variable that holds our container, and making sure that the container is exposed publicly, and we’re doing that by using a property.
Now we move into how our enemy will detect and avoid our lasers.
private float _avoidDistance = 3.0f;
private void CalculateMovement()
{
switch (_type)
{
//other cases
case EnemyType.Evasive:
Vector3 _enemyMoveDirection = Vector3.down;
bool _foundLaser = false;
LaserBehaviour[] _trackLasers = SpawnManager.Instance.PlayerLaserContainer.GetComponentsInChildren<LaserBehaviour>();
for (int i = 0; i < _trackLasers.Length; i++)
{
if (_trackLasers[i] != null && _trackLasers[i].gameObject != null && _trackLasers[i].gameObject.activeSelf)
{
Vector3 _directionToLaser = _trackLasers[i].transform.position - transform.position;
if (Mathf.Abs(_directionToLaser.magnitude) < _avoidDistance)
{
_enemyMoveDirection = -_directionToLaser;
_foundLaser = true;
}
}
}
if(_foundLaser)
transform.Translate(_enemySpeed / 2f * Time.deltaTime * _enemyMoveDirection);
else
transform.Translate(_enemySpeed * Time.deltaTime * _enemyMoveDirection);
break;
default:
break;
}
TeleportNewPosition();
}
Lets break down everything we are doing here:
_enemyMoveDirection: We create a Vector3.down, indicating that it should move downwards by default, in case no laser is found.
_foundLaser: This is our bool to indicate if a laser has been found. If it has not, we will move downwards normally.
_trackLasers: This is our variable that holds an array of all lasers found within the container from our Singleton class SpawnManager.
Then we loop through all items in the array, and check if they are not null and active in scene.
_directionToLaser: It calculates the direction from the enemy to the laser.
Then we check if the distance to the laser is within the specified _avoidDistance.
If the distance to the laser is within the specified _avoidDistance, it updates our _enemyMoveDirection to move away from the laser and sets _foundLaser to true.
And lastly, we check if we have found a laser to avoid. If we did, we move away from it, at half speed. Otherwise, we just normally move downwards.

Now as we can see, when we shoot a laser towards our Evasive Enemy, he will try to avoid it at all costs. Good luck out there!