Creating an Ammo System
Objective: Learn how to create an ammo system for our player.
So our player can easily destroy our enemies, as spamming his lasers have no drawback. How about we add some difficulty for our player ?
In order to create such system, we need a way to check for our Ammo. So first things first, we are going to our variable that holds the ammo for our player, and the UI Part that will display it on the screen.
private int _ammoCount = 15;
This will be our variable for ammo. Now in our UI Manager we create our Text object.
[SerializeField] private TextMeshProUGUI _ammoText;
And then we just make sure to assign it in the Inspector, and fix its position.
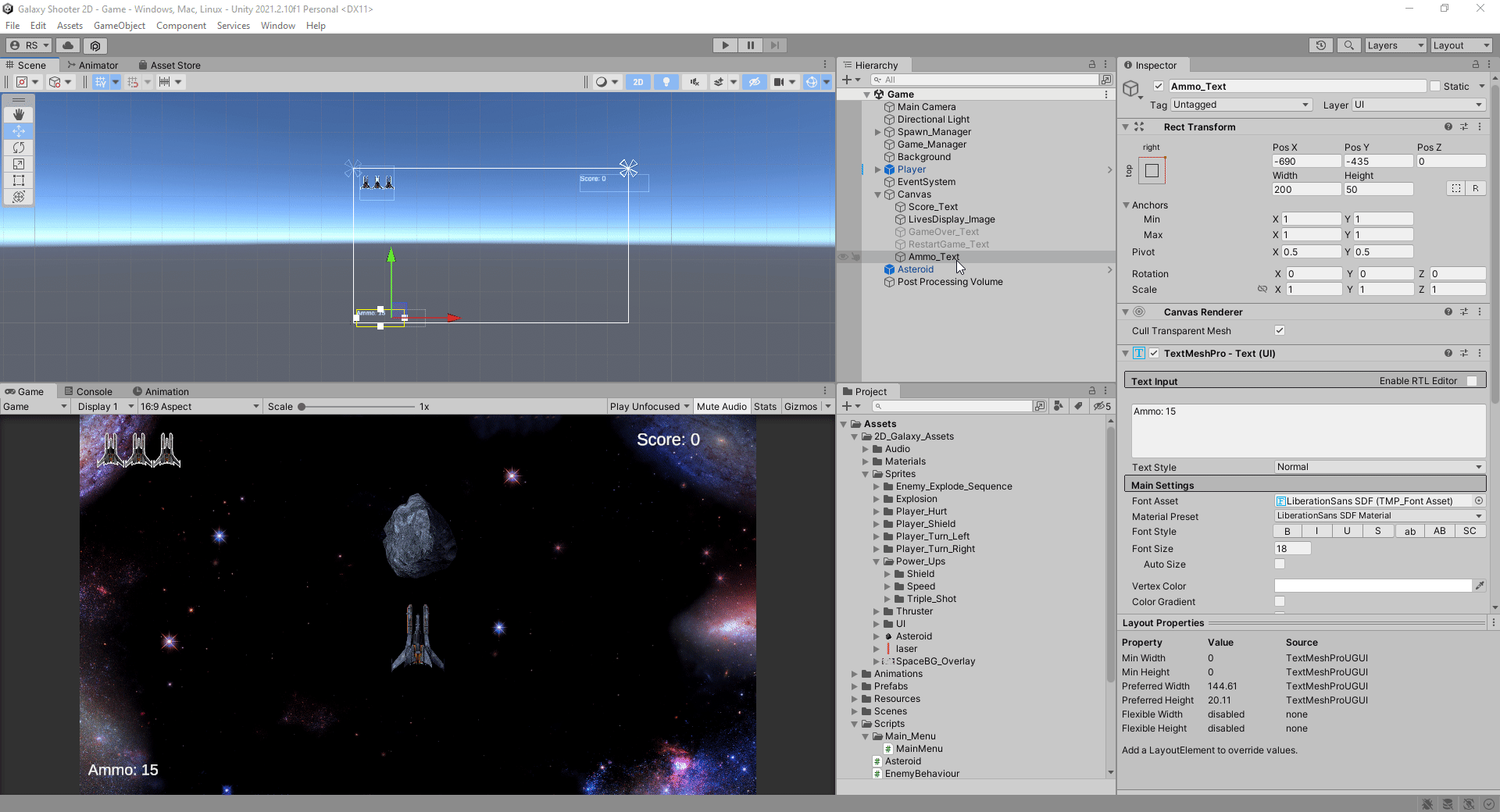
So how do we go about checking for our ammo usage ? Well, we have a method that fires the laser for the player, right ? Before we are allow to fire, we need to check if we have enough ammo, and if that is the case, we can fire.
private void Update()
{
float moveX = Input.GetAxis("Horizontal");
float moveY = Input.GetAxis("Vertical");
_direction.Set(moveX, moveY, 0);
MovePlayer(_direction);
if (Input.GetKeyDown(KeyCode.Space) && _canFire <= Time.time)
{
if(_ammoCount > 0)
{
_ammoCount--;
_uiManager.UpdateAmmoAmount(_ammoCount);
FireLaser();
}
}
}
So when our player presses space, we check if the player has enough ammo to fire our laser. If that is the case, we deduct one ammo from the player and fire the laser. Otherwise, we don’t fire at all.
While doing that, we also tell our UI Manager to update the ammo display.
public void UpdateAmmoAmount(int ammo)
{
_ammoText.text = "Ammo: " + ammo.ToString();
}
Now with our UI Manager properly displaying the information, lets see how the Ammo System is working.

In our next tutorial we will check on how to create a Powerup that will grant Ammo back to our player!